Using Function Import in an OData Service
Using Function Import in an OData Service
Introduction
In the OData service development toolkit within the SAP system, there's an interesting feature that I’ve rarely had the opportunity to work with—Function Import. This post focuses on exploring this feature.
Theoretical Background
Function Import is a service operation (essentially a request) executed on the backend system. Using a function import instead of a conventional OData request may be justified when the operation cannot be adequately described using the standard CRUD model. Below are some examples of when Function Import might be appropriate:
- Starting or stopping a workflow;
- Querying custom tables or external systems;
- Fetching additional data not currently included in the data model;
- And other specialized operations.
See also: Function Imports
The Open Data Protocol (OData) includes standard CRUD (Create, Retrieve, Update, and Delete) operations that map to the HTTP methods POST, GET, PUT/MERGE, and DELETE. In addition, OData supports further service operations (function imports) that can be invoked by the HTTP methods GET or POST for anything that cannot be mapped to the standard CRUD operations. You can implement such additional service operations in the Service Builder by creating function imports within your data model.
Creating a Function Import for an OData Service
In the structure of your OData service (transaction SEGW), right-click on the Data Model node and select:Create -> Function Import
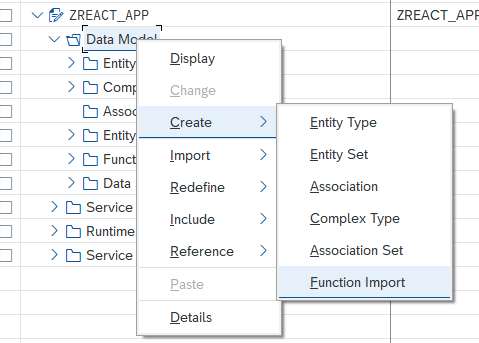
Enter a name for the new function.
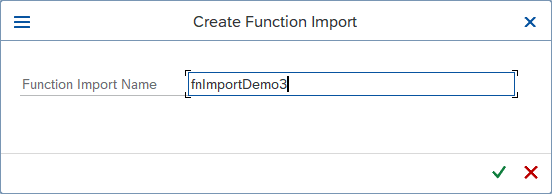
Define the return data type and select an appropriate HTTP method.
See also: Function Imports
In the Return Type column, enter the name of the complex type or entity type you want the function import to return. You can use the input help to select an existing complex or entity type from your project. If the input help is empty, you must first create the relevant complex type or entity type in your project. If you selected No Return this field is non-editable

Define the input parameters for the Function Import.
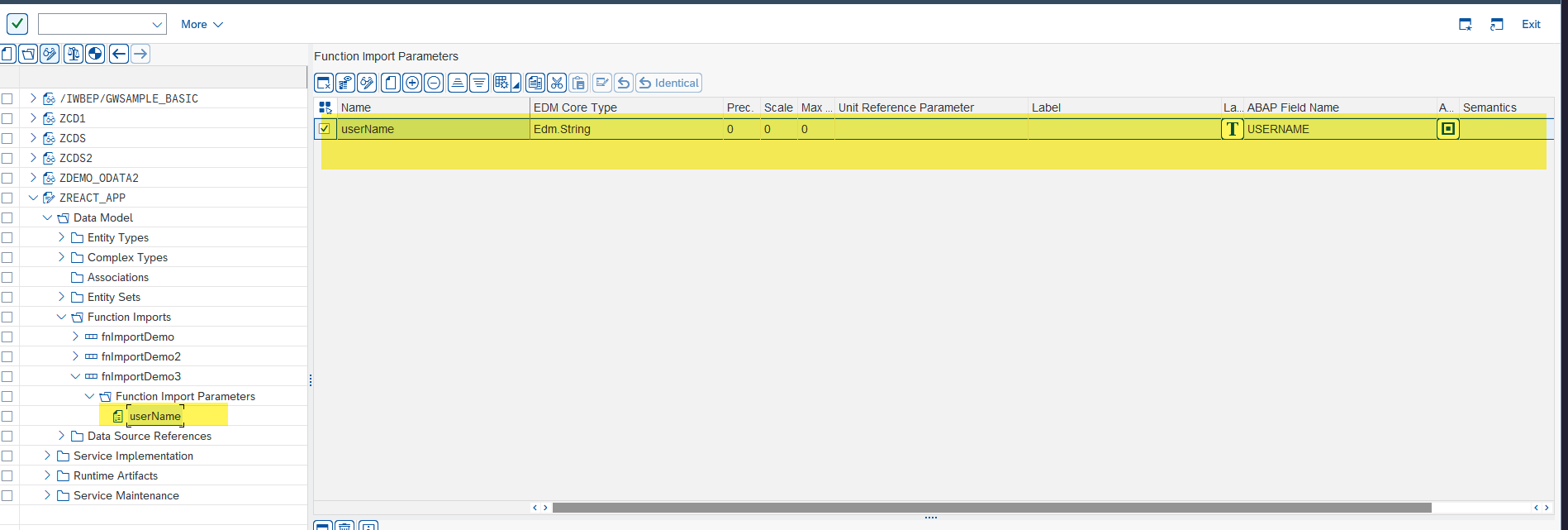
Modifying the _DPC_EXT
Class of the OData Service
In the DPC_EXT
class of your OData service, override the EXECUTE_ACTION
method available in the /IWBEP/IF_MGW_APPL_SRV_RUNTIME
interface.
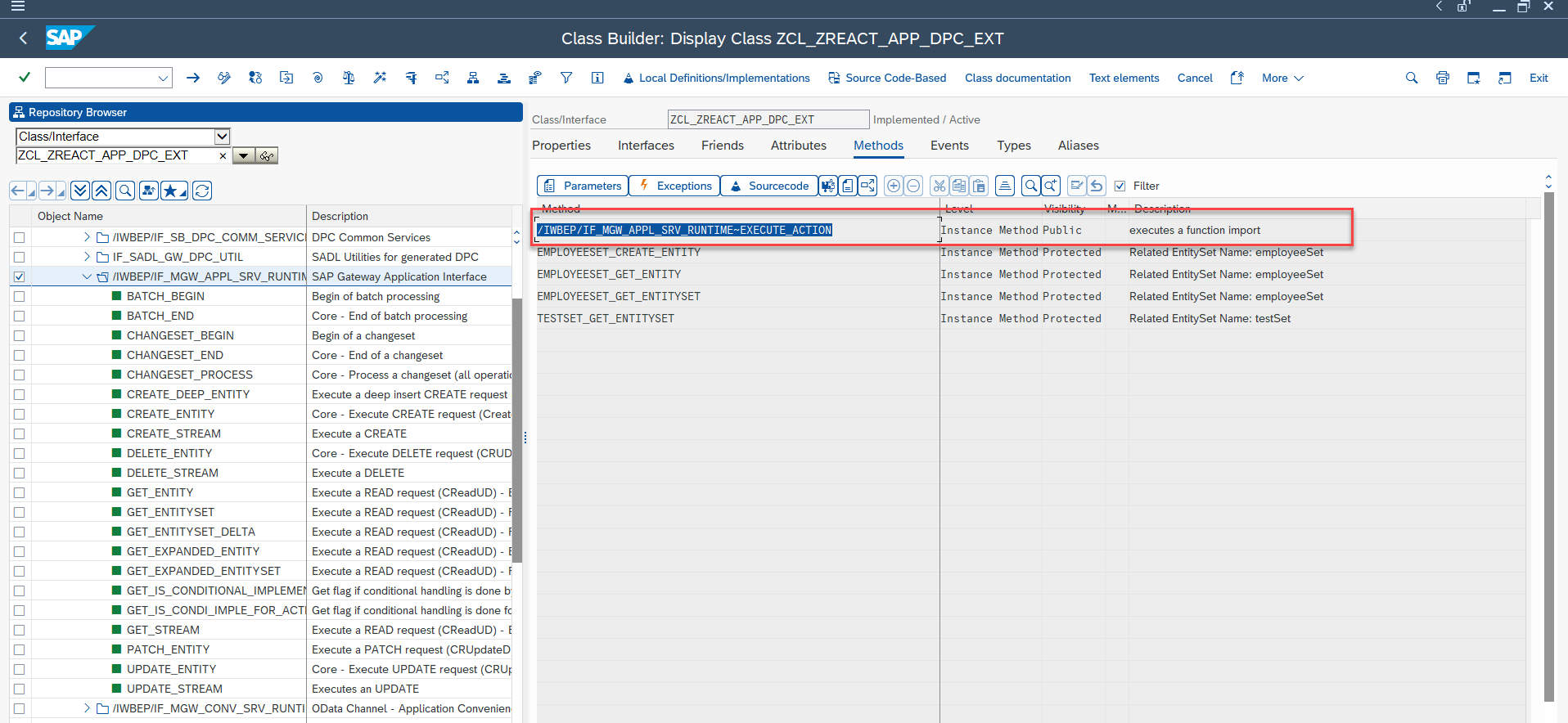
How to Call a Function Import
You can call a Function Import by referencing its name in the OData service and providing the required parameters:
/sap/opu/odata/SAP/<your_OData_service_name>/<FunctionImport_name>?<parameter_name>='value'
Example:
/sap/opu/odata/SAP/ZREACT_APP_SRV/fnImportDemo3?userName='developer'
Example: Task Definition
Create a Function Import to check if a user has the required authorizations. The result should populate an entitySet
according to its structure.
See also: How to Create a Custom Authorization Object?
Example: Task Implementation (1)
Function Import created with one input parameter.
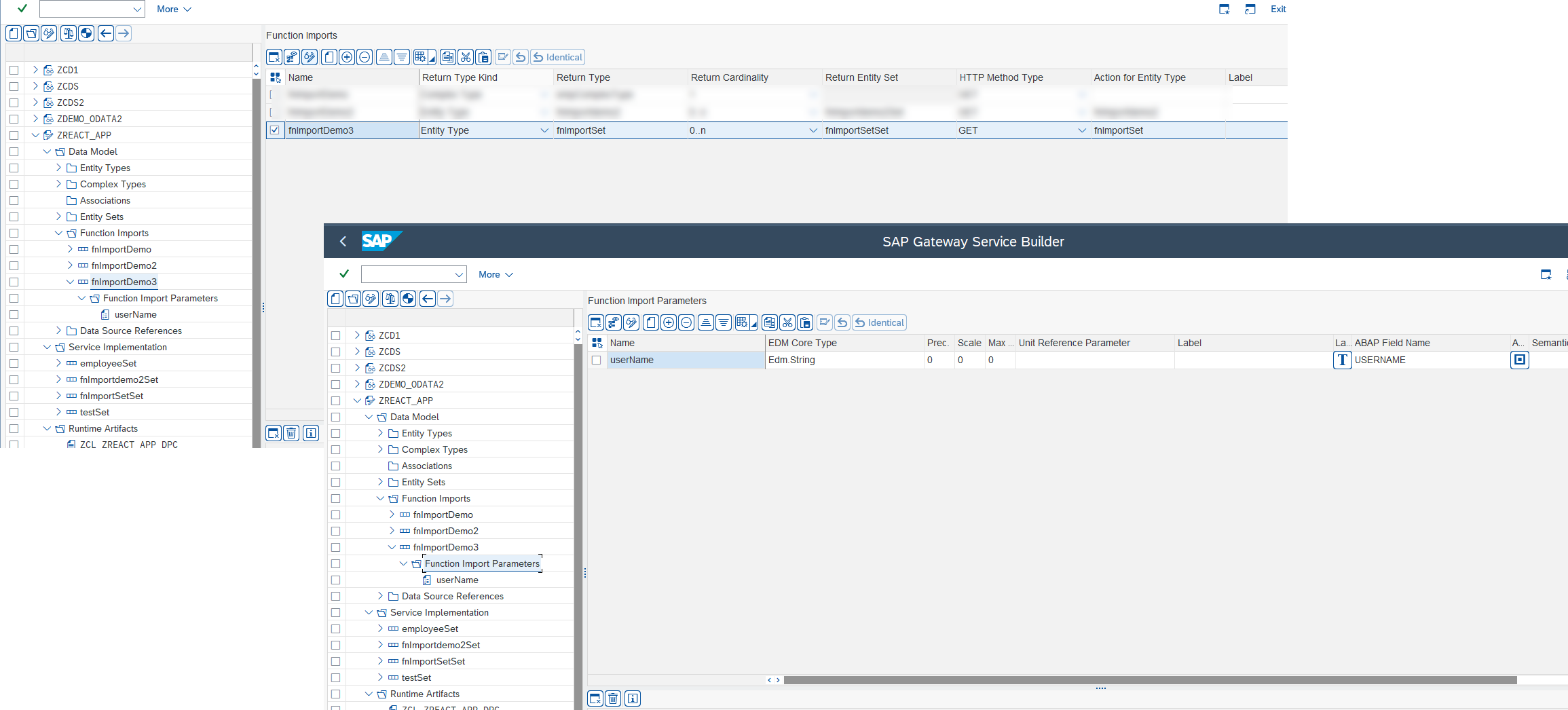
Example: Task Implementation (2)
Structure of the entitySet
to be populated as a result of the Function Import call.
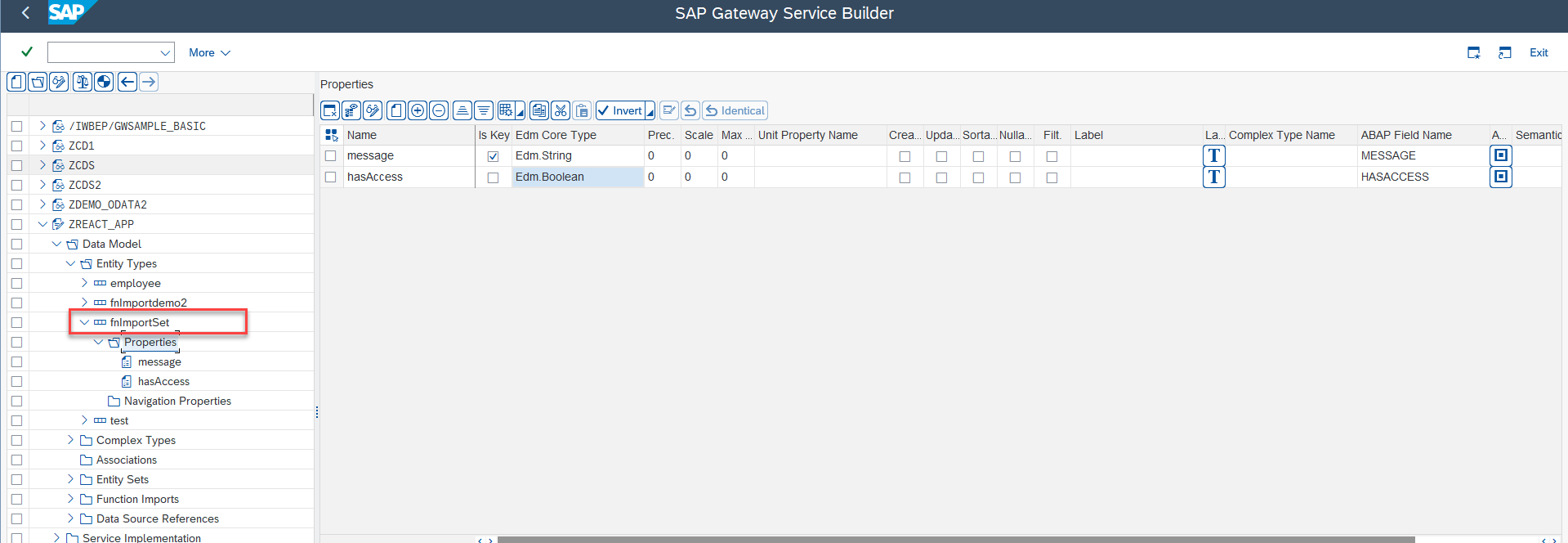
Example: Task Implementation (3)
Source code of the Function Import logic.
METHOD /iwbep/if_mgw_appl_srv_runtime~execute_action.
DATA: ls_fnset TYPE zcl_zreact_app_mpc=>ts_fnimportset,
et_fnset TYPE zcl_zreact_app_mpc=>tt_fnimportset,
lv_user TYPE sy-uname.
DATA(lv_fnName) = io_tech_request_context->get_function_import_name( ).
CASE lv_fnName.
WHEN 'fnImportDemo3'.
LOOP AT it_parameter ASSIGNING FIELD-SYMBOL(<fs>).
IF <fs> IS ASSIGNED.
IF <fs>-name EQ 'userName'.
lv_user = <fs>-value.
ENDIF.
ENDIF.
ENDLOOP.
TRANSLATE lv_user TO UPPER CASE.
AUTHORITY-CHECK OBJECT 'ZBTN' FOR USER lv_user ID 'ACTVT' FIELD '02' .
ls_fnset-hasAccess = abap_false.
IF sy-subrc EQ 0.
ls_fnset-message = lv_user && ' has access'.
ls_fnset-hasAccess = abap_true.
ELSE.
ls_fnset-message = lv_user && ' has no access'.
ENDIF.
APPEND ls_fnset TO et_fnset.
copy_data_to_ref( EXPORTING is_data = et_fnset
CHANGING cr_data = er_data ).
ENDCASE.
ENDMETHOD.
Example: Calling the Function Import (4)
The following video shows the steps to call the Function Import and adjust the value passed as a parameter to the custom authorization object.
Example: Using Function Import in a SAPUI5 Application (5)
The next video shows a SAPUI5 application that calls the Function Import from the OData service. The result of the call is shown using a MessageToast.
See also: callFunction